How to Perform CRUD Operations using React, React Hooks, and Axios | React JS update data with React Hook useState, useEffect
If you
start working with React JS, it can be quite difficult to understand and implement
API Requests with node JS express or php api or other API.
So in this short article, we'll
learn how it all works by implementing CRUD Operations using React, React
Hooks, React Router, Usestate, UseEffect and Axios.
Let's start to do this ….
How to
Install Node and npm
First of all, let's install Node
in our system. We'll mainly use it to execute our JavaScript code.
To download Node, go
to https://nodejs.org/en/.
You'll also need the
node package manager, or npm, which is built on Node. You can use it to install
packages for your JavaScript apps. Fortunately it comes with Node, so you don't
need to download it separately.
Once both of them are
finished, open your terminal or command prompt and type node -v. This checks which version of Node you have.
How to Create your React
Application
To create your React application, type npx
create-react-app <Your app name>
in your
terminal, or npx create-react-appreact-crud
in
this case.
You'll
see that the packages are being installed.
Once the packages are done, go
into the project folder and type npm start
.
You'll
see the default React template, like this:
React JS Template is look Like this.
How to Install the Semantic UI Package for React
Let's install the Semantic UI React package in our project. Semantic UI is an UI library made for React that has pre-built UI components like tables, buttons, and many features.
You can install it using one of the commands below, depending on your package manager.
yarn add semantic-ui-react semantic-ui-css
npm install semantic-ui-react semantic-ui-css
Also, import the library in your app's main entry file, which is index.js.
import 'semantic-ui-css/semantic.min.css'
How to Build your CRUD Application
Now, let's start building our CRUD Application using React.
First, we'll add a heading to our application.
In our app.js file, add a heading like this:
import './App.css';
function App() {
return (
<div>
React Crud Operations
</div>
);
}
export default App;
Now, let's make sure it's in the center.
Give the parent div a classname of main. And in the App.css file, we will use Flexbox to center the header.
import './App.css';
function App() {
return (
<div className="main">
React Crud Operations
</div>
);
}
export default App;
.main{
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
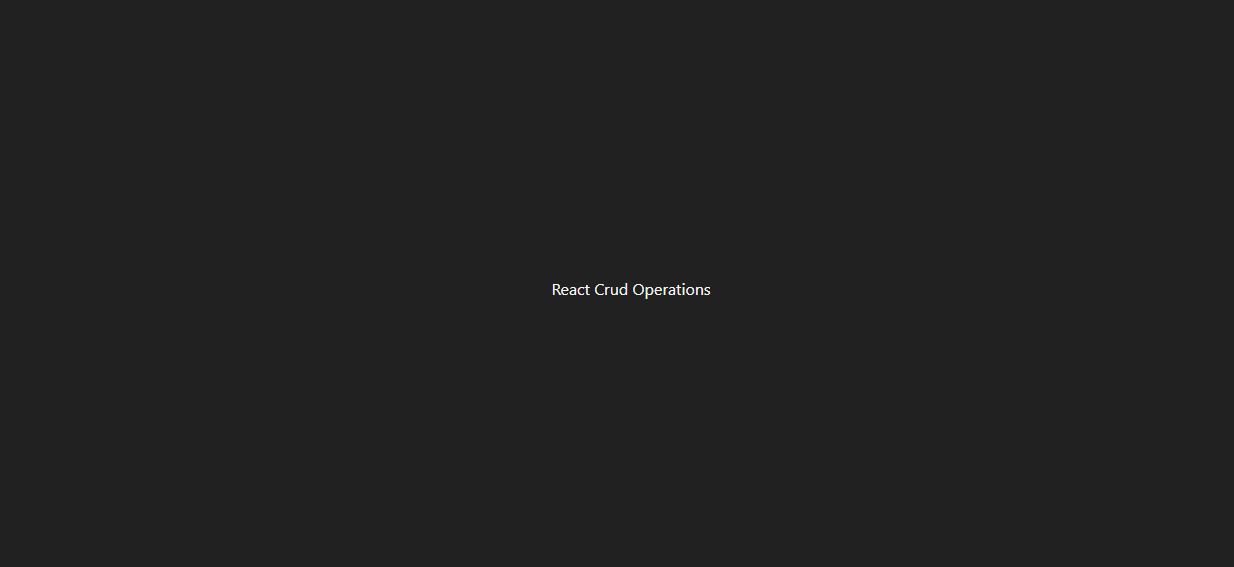